Clean Code: Tips for Writing Code that is Easy to Read, Understand, and Maintain
Clean code is code that is easy to read, understand, and maintain. It follows certain rules and principles to ensure that it is of high quality.
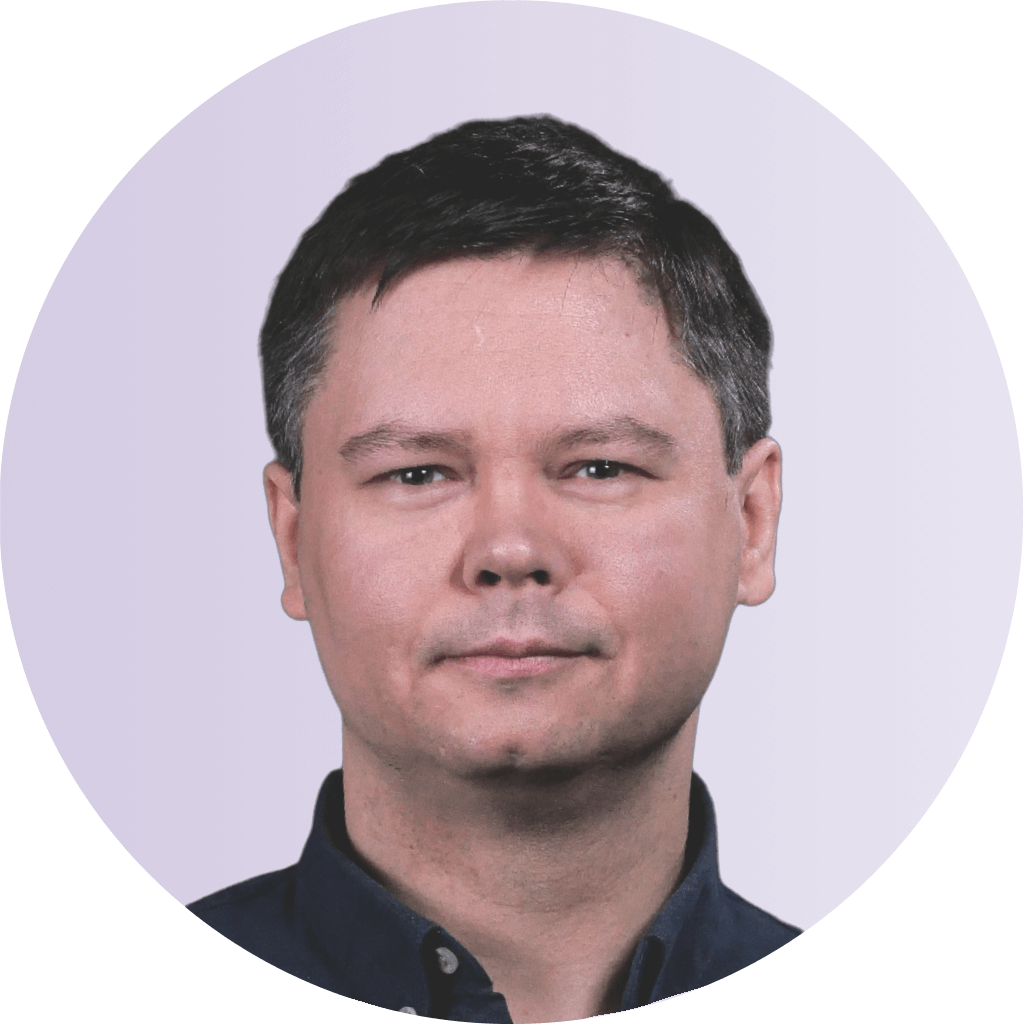
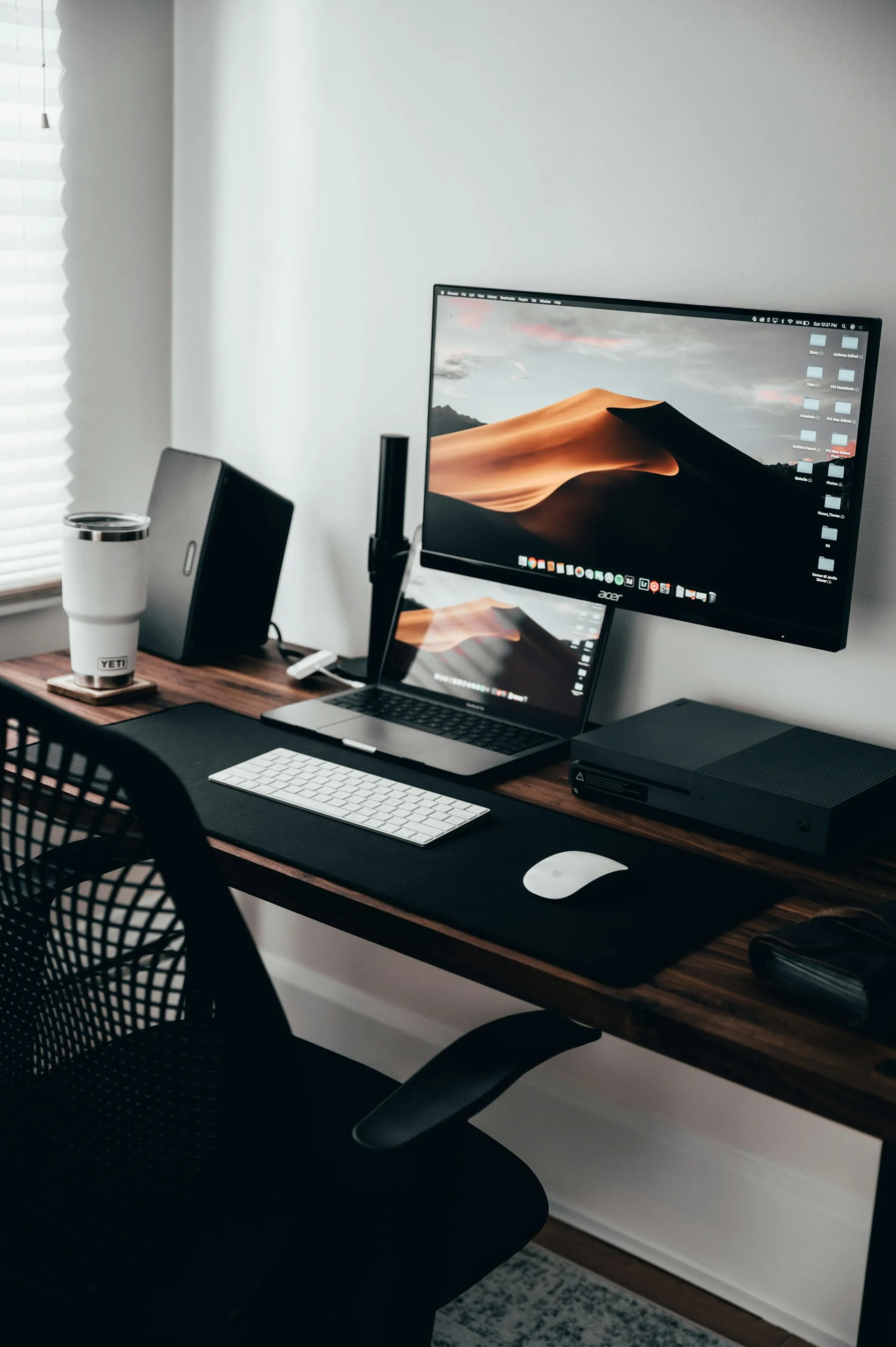
Clean Code is a set of rules and principles designed to help you write code that is easy to read, understand, and maintain. Here is a list of things you should avoid or improve in your code.
Bad Naming
How often have you seen poorly named variables, functions, and classes? I’ve seen them quite a lot. Good naming makes code easier to read and understand, reducing the need for comments. Here’s a general rule of thumb for naming variables, methods/functions, and classes:
- Variable: noun, adjective (“user”)
- Method/Function: verb (“GetUser”)
- Class: noun (“User”)
Apply these rules to any programming language, and if in doubt, follow the language conventions.
Comments
Some love them; others hate them. They can be helpful or misleading. Good code doesn’t need comments to explain how something works. Use comments to explain why you did something or highlight something that isn’t obvious.
Code Repetition
Repeated code is often a mistake made by beginners or those on tight deadlines. While some duplication might be acceptable, generally, you should aim to have code in a single place to make changes easier. However, don’t follow this rule blindly!
Zombie Code
There’s nothing worse than commented-out code. Why was it commented out? Should it do something? It creates too many questions and makes you wonder about its purpose. Similarly, unreachable or unused code should be deleted. You can always find it in source control later.
Long Methods
What makes a method too long? A general rule of thumb is that it’s too long if it doesn’t fit on the screen. Even if your 100-line method fits on a high-resolution screen, it’s usually doing too many things at once.
Why are long methods bad?
- They often do too many things at once
- They are hard to read and understand
- They are harder to test
You can often split one big method into smaller methods focusing on specific tasks. Long methods also tend to have many arguments in their signatures.
Methods with Too Many Arguments
Some say a method shouldn’t have more than 3-4 arguments. Too many arguments often indicate that a method is doing more than it should. You can group arguments and introduce a new type (class or record). For instance, instead of passing “first name,” “last name,” “date of birth,” and “email,” create a new Person
type with those properties.
Nested (Arrow) Code
Nested code is hard to read and often performs poorly. It’s called arrow code because the indentation often resembles an arrow’s tip.
What can you do about it?
- Simplify the code
- Use guard clauses and early returns
- For long loop bodies, extract them into separate methods
Nested loops are the worst offenders, often with long bodies that make the code hard to read and understand. Keep in mind the previous tips when refactoring long loops.
God Classes
Classes with very low cohesion try to do too many things at once. The solution is to split the class up. Don’t try to cheat with partial classes.
How Do We End Up with Bad Code in the First Place?
People don’t write bad code intentionally. Here are some common reasons:
- Lack of mentorship
- No code reviews
- Lack of skills
- “Just get it done” culture
- Deadlines
Mentoring is effective but time-consuming, especially for junior developers. If your company or team doesn’t use code reviews (PRs, pair programming), consider starting. Trusting people to write good code is nice, but having more than one pair of eyes on the code is even better.
Some companies prioritize profits over quality. Tight deadlines and small budgets might force developers to make unpopular choices.
Every developer should be a responsible individual who cares about their craft. As a developer, you should:
- Apply engineering principles
- Invest in knowledge
- Not be afraid to ask for help
- Learn from mistakes
- Think critically
Becoming proficient in any craft takes time, and software development is no different. Don’t worry – with persistence, you can do it.